Creating a WordWriter Template
|
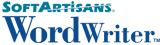 |
Creating a Merge Field
A WordWriter template is a Microsoft Word file that contains merge fields.
A merge field displays a data source field name (for example, a database column
name).
WordWriter's setDataSource
method binds the merge fields to a data source, which may be an array or a database
table record. WordWriter's
process method enters values
from the data source in the template's merge fields.
- In Microsoft Word, open or create a document to use as
your WordWriter template.
- From the Insert menu, select Field... to open
the Field dialog.
- From the Field names list, select MergeField.
- In the Field name text box, enter a name for the merge
field. For example, enter ProductName.
- Optional: From the Format list, select a display format.
- Optional: In Field Options, check Text to be
inserted before and enter text to insert before the merge field.
- Optional: In Field Options, check Text to be
inserted after and enter text to insert after the merge field.
WordWriter 1.0 does not support the following formatting
options: Mapped field and Vertical formatting. |
|
The Field Dialog in Microsoft Word 2002 (XP) |
Top
Example: Creating a Template Envelope
To create a template envelope:
- Open Microsoft Word.
- To display the Mail Merge toolbar, open the View
menu and select Toolbars -> Mail Merge.
- Click the Main document setup icon.

- Select Envelopes.
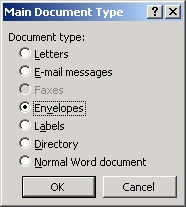
- In the Envelope Options dialog, select envelope size,
address fonts, and printing options.
- Within the main document (the envelope), in the delivery and return address boxes,
enter merge fields. For instructions, see Creating a Merge Field.
The following code example uses WordWriter to open a template envelope,
set a data source, populate the merge fields, and generate a new envelope.
The template envelope contains merge fields for the delivery name and address and
the return name and address. The WordWriter code uses an array as the data source and
gets the array values from a Web form.
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
import com.softartisans.wordwriter.WordTemplate;
public class WwServlet extends HttpServlet
{
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException
{
//--- Declare the WordTemplate object.
WordTemplate oWW = null;
//--- Path to the template file, relative to the Servlet context root.
String templateFile = "/Intermediate/Envelope/Envelope10Template.doc";
//--- Form the array of mergefield names.
String[] arrNames = {"RtnName", "RtnStreet", "RtnCityStateZip", "RcpName",
"RcpStreet", "RcpCityStateZip"};
//--- Form the value array. The value of the elements in this array contain
//--- info supplied in the Web form. These values represent the data that
//--- will be dynamically populated in the template file.
Object[] arrValues = new Object[6];
arrValues[0] = request.getParameter("txtRtnAddrName");
arrValues[1] = request.getParameter("txtRtnAddrStreet");
arrValues[2] = request.getParameter("txtRtnAddrCityStateZip");
arrValues[3] = request.getParameter("txtRcpAddrName");
arrValues[4] = request.getParameter("txtRcpAddrStreet");
arrValues[5] = request.getParameter("txtRcpAddrCityStateZip");
//--- Declare the InputStream we will use to open the template.
InputStream iStream = null;
try
{
//--- Instantiate the WordTemplate object.
oWW = new WordTemplate();
//--- Open Word template file as a resource: have to do it
//--- this way to work from unexpanded WAR files. For greatest
//--- efficiency and reliability, use a BufferedInputStream.
ServletContext ctx = this.getServletContext();
iStream = new BufferedInputStream(ctx.getResourceAsStream(templateFile));
//--- Open the template file.
oWW.open(iStream);
//--- Set the datasource with the name and value arrays defined above.
oWW.setDataSource(arrValues, arrNames);
//--- Process the template.
oWW.process();
//--- Begin streaming the processed template.
oWW.save(response, "EnvelopeOutput.doc", false);
}
catch(Exception ex)
{
//--- Catch any exceptions thrown by WordWriter.
response.resetBuffer();
response.getWriter().println("<B>An exception has occurred</B><BR /> " + String.valueOf(ex));
}
finally
{
//--- Close the template input stream
if(iStream!=null)
iStream.close();
}
}
}
|
Top
Example: A Fax Cover Template
To create a fax cover template using one of Microsoft Word's available templates:
- In Microsoft Word, open the File menu, and select New...
- From the New Document window, select General Templates...
- In the Templates dialog, select the Letters & Faxes tab.
- Select one of the available fax templates.
- Insert merge fields in the template.
The following code uses WordWriter to open a fax cover template,
set a data source, populate the merge fields, and generate a new fax cover document.
The template contains the merge fields ToName, FromName, FaxNumber, PhoneNumber,
Subject, PageCount, Comments, chkUrgent, chkReview, and chkComment. The WordWriter code uses
an array as the data source and gets the array values from a Web form.
Check boxes in the Web form provide the
values for chkUrgent, chkReview, and chkComment. If a box is checked, "X" will be entered
in the data source value array and displayed in the generated file. If a box is not
checked, an empty string will be entered in the value array and nothing will be displayed
by the field name (for example, by "Urgent") in the generated file.
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
import com.softartisans.wordwriter.WordTemplate;
public class WwServlet extends HttpServlet
{
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException
{
//--- Declare WordTemplate.
WordTemplate oWW = null;
//--- Path to the template file, relative to the Servlet context root.
String templateFile = "/Intermediate/FaxCover/FaxCoverTemplate.doc";
//--- Form the array of mergefield names.
String[] arrNames = {"ToName", "FromName", "Company", "FaxNumber",
"PhoneNumber", "Subject", "PageCount", "Comments", "ChkUrgent",
"ChkReview", "ChkComment"};
//--- The value array. The value of the elements in this array contain
//--- info supplied in the Web form. These values represent the data that
//--- will be dynamically populated in the template file
Object[] arrValues = new Object[11];
arrValues[0] = request.getParameter("txtToName");
arrValues[1] = request.getParameter("txtFromName");
arrValues[2] = request.getParameter("txtCompany");
arrValues[3] = request.getParameter("txtFaxNumber");
arrValues[4] = request.getParameter("txtPhoneNumber");
arrValues[5] = request.getParameter("txtSubject");
arrValues[6] = request.getParameter("txtPageCount");
arrValues[7] = request.getParameter("txtComments");
//--- For the check fields, we'll put an X in the document if checked,
//--- and an empty string is not.
arrValues[8] = request.getParameter("chkUrgent")!=null ? "X" : "";
arrValues[9] = request.getParameter("chkReview")!=null ? "X" : "";
arrValues[10] = request.getParameter("chkComment")!=null ? "X" : "";
//--- Declare the InputStream we will use to open the template.
InputStream iStream = null;
try
{
//--- Instantiate the WordTemplate object.
oWW = new WordTemplate();
//--- Open Word template file as a resource: have to do it this way to work
//--- from unexpanded WAR files. For greatest efficienty and reliability,
//--- use a BufferedInputStream.
ServletContext ctx = this.getServletContext();
iStream = new BufferedInputStream(ctx.getResourceAsStream(templateFile));
//--- Open the template file.
oWW.open(iStream);
//--- Set the datasource with the name and value arrays defined above.
oWW.setDataSource(arrValues, arrNames);
//--- Process the template.
oWW.process();
//--- Begin streaming the processed template.
oWW.save(response, "FaxCoverOutput.doc", false);
}
catch(Exception ex)
{
//--- Catch any exceptions thrown by WordWriter.
response.resetBuffer();
response.getWriter().println("<B>An exception has occurred</B><BR /> " + String.valueOf(ex));
}
finally
{
//--- Close the template input stream.
if(iStream!=null)
iStream.close();
}
}
}
|
Top
Example: A Form Letter Template
The following code example uses WordWriter
to open a template form letter, set a data source, populate the merge fields,
and generate a new file. The template form letter contains merge fields for
recipient name and address and author name and title. The WordWriter code uses
an array as the data source and gets the array values from a Web form.
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
import com.softartisans.wordwriter.WordTemplate;
public class WwServlet extends HttpServlet
{
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException
{
//--- Declare the WordTemplate object.
WordTemplate oWW = null;
//--- Path to the template file, relative to the Servlet context root.
String templateFile = "/Intermediate/FormLetter/FormLetterTemplate.doc";
//--- Copy values from the form into local string variables.
String recipName = request.getParameter("txtRecipientName");
String recipStreetAddr = request.getParameter("txtRecipientStreetAddr");
String recipCity = request.getParameter("txtRecipientCity");
String recipState = request.getParameter("txtRecipientState");
String recipZip = request.getParameter("txtRecipientZip");
String authName = request.getParameter("txtAuthorName");
String authTitle = request.getParameter("txtAuthorTitle");
//--- Form the required WordWriter name array. The elements in
//--- this array correspond to the names of the merge fields
//--- in the template, and each element's array index should
//--- correpond to an element in the value array.
String[] arrNames = {"RecipientName", "RecipientStreetAddr",
"RecipientCity", "RecipientState",
"RecipientZip", "AuthorName", "AuthorTitle"};
//--- The value array. The value of the elements in this array
//--- contain info supplied in the Web form. These values represent
//--- the data that will be dynamically populated in the template file.
Object[] arrValues = {recipName, recipStreetAddr, recipCity, recipState,
recipZip, authName, authTitle};
//--- Declare the InputStream we will use to open the template.
InputStream iStream = null;
try
{
//--- Instantiate the WordTemplate object.
oWW = new WordTemplate();
//--- Open Word template file as a resource: have to do it this way to work
//--- from unexpanded WAR files.
//--- For greatest efficienty and reliability, use a BufferedInputStream.
ServletContext ctx = this.getServletContext();
iStream = new BufferedInputStream(ctx.getResourceAsStream(templateFile));
//--- Open the template file.
oWW.open(iStream);
//--- Set the datasource with the name and value arrays defined abov.
oWW.setDataSource(arrValues, arrNames);
//--- Process the template.
oWW.process();
//--- Begin streaming the processed template.
oWW.save(response, "FormLetterOutput.doc", false);
}
catch(Exception ex)
{
//--- Catch any exceptions thrown by WordWriter.
response.resetBuffer();
response.getWriter().println("<B>An exception has occurred</B><BR />" + String.valueOf(ex));
}
finally
{
//--- Close the template input stream.
if(iStream!=null)
iStream.close();
}
}
}
|
Top
Copyright © 2003, SoftArtisans, Inc.